数据模板DataTemplate - (二十七)
这里提供一种DataTemplate使用方法,还有如何获取ListView当前选中项:
运行效果如下:
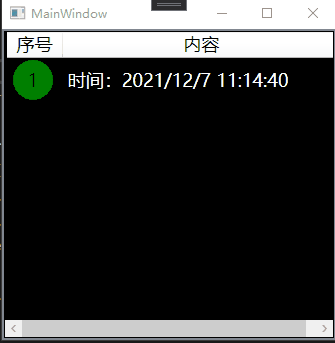
MainWindow.xaml 代码如下:
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="350">
<Grid>
<!-- 分两列 -->
<Grid.ColumnDefinitions>
<ColumnDefinition Width="55" />
<ColumnDefinition Width="*"/>
</Grid.ColumnDefinitions>
<Grid Grid.Column="0" x:Name="column1"/>
<Grid Grid.Column="1" x:Name="column2"/>
<ListView Name="MyListView" BorderThickness="2" Grid.ColumnSpan="2" Background="Black" FontSize="18" >
<ListView.View>
<!-- AllowsColumnReorder:是否允许排序 -->
<GridView AllowsColumnReorder="False">
<GridViewColumn Header="序号" Width="{Binding ElementName=column1,Path=ActualWidth}">
<GridViewColumn.CellTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal">
<!-- Ellipse 画圆 -->
<Ellipse Name="ep" Width="40" Height="40" Fill="Gray" DataContext="111" />
<!-- RowIndex 是序号 -->
<TextBlock Grid.Column="0" Margin="-40,0,0,0" Foreground="Black" VerticalAlignment="Center"
HorizontalAlignment="Center" FontSize="18" Text="{Binding RowIndex }" ></TextBlock>
</StackPanel>
<!-- Status 是状态,根据不同的Value值设置圆(ep)的不同颜色 -->
<!-- DataTrigger 是数据触发器 -->
<DataTemplate.Triggers>
<DataTrigger Binding="{Binding Status}" Value="0">
<Setter Property="Fill" Value="blue" TargetName="ep"/>
</DataTrigger>
<DataTrigger Binding="{Binding Status}" Value="1">
<Setter Property="Fill" Value="Red" TargetName="ep"/>
</DataTrigger>
<DataTrigger Binding="{Binding Status}" Value="2">
<Setter Property="Fill" Value="Green" TargetName="ep"/>
</DataTrigger>
</DataTemplate.Triggers>
</DataTemplate>
</GridViewColumn.CellTemplate>
</GridViewColumn>
<GridViewColumn Header="内容" Width="{Binding ElementName=column2,Path=ActualWidth}" x:Name="MyText">
<GridViewColumn.CellTemplate>
<DataTemplate>
<TextBlock Grid.Column="0" TextWrapping="Wrap" Foreground="White" VerticalAlignment="Center"
HorizontalAlignment="Center" FontSize="18" Text="{Binding Text }" ></TextBlock>
</DataTemplate>
</GridViewColumn.CellTemplate>
</GridViewColumn>
</GridView>
</ListView.View>
</ListView>
</Grid>
</Window>
MainWindow.xaml.cs 代码如下:
using System;
using System.Collections.ObjectModel;
using System.ComponentModel;
using System.Threading;
using System.Windows;
namespace WpfApp
{
/// <summary>
/// MainWindow.xaml 的交互逻辑
/// </summary>
public partial class MainWindow : Window
{
/// <summary>
/// 信息
/// </summary>
public ObservableCollection<CurrenTrayInfoList> CurrenTrayInfoListOne { get; set; } = new ObservableCollection<CurrenTrayInfoList>();
public MainWindow()
{
InitializeComponent();
// 信息
MyListView.ItemsSource = CurrenTrayInfoListOne;
// 启动线程
new Thread(ThreadData) { IsBackground = true }.Start();
}
private void ThreadData()
{
Thread.Sleep(3000);
Random rd = new Random();
for (int i = 1; i <= 5; i++)
{
CurrenTrayInfoList currentTrayEntity = new CurrenTrayInfoList();
currentTrayEntity.RowIndex = i;
currentTrayEntity.Text = $"时间:{DateTime.Now}";
currentTrayEntity.Status = rd.Next(4);
Dispatcher.BeginInvoke((Action)delegate ()
{
CurrenTrayInfoListOne.Add(currentTrayEntity);
});
Thread.Sleep(1000);
}
// 获取选中状态
while (true)
{
Dispatcher.BeginInvoke((Action)delegate ()
{
CurrenTrayInfoList c = MyListView.SelectedItem as CurrenTrayInfoList;
if (c != null) MyText.Header = c.RowIndex + ":" + c.Text;
});
Thread.Sleep(100);
}
}
public class CurrenTrayInfoList : ModelBase
{
private int _rowindex;
private string _text;
private int _status;
/// <summary>
/// 序号
/// </summary>
public int RowIndex
{
set
{
_rowindex = value;
this.OnPropertyChanged("RowIndex");
}
get { return _rowindex; }
}
/// <summary>
/// Text
/// </summary>
public string Text
{
set
{
_text = value;
this.OnPropertyChanged("Text");
}
get { return _text; }
}
/// <summary>
/// 状态
/// </summary>
public int Status
{
set
{
_status = value;
this.OnPropertyChanged("Status");
}
get { return _status; }
}
}
[Serializable]
public abstract class ModelBase : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged(string propertyName)
{
if (propertyName != null)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
}
}
}