Polygon绘制多边形 - (二十五)
运行效果如下:
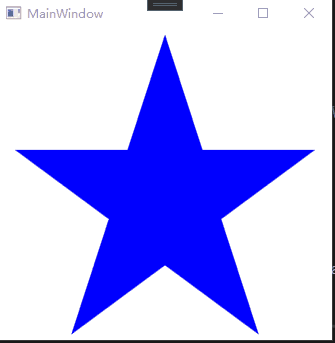
MainWindow.xaml 代码如下:
<Window x:Class="WpfApp.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="350">
<Grid>
<Polygon Margin="2"
Fill="Red" Stroke="Black" StrokeThickness="0"
StrokeLineJoin="Round" Width="300" Height="300"
Stretch="Fill"
Points="9,2 11,7 17,7 12,10 14,15 9,12 4,15 6,10 1,7 7,7"
Name="Star"/>
</Grid>
</Window>
MainWindow.xaml.cs 代码如下:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Threading;
using System.Windows;
using System.Windows.Data;
using System.Windows.Shapes;
namespace WpfApp
{
/// <summary>
/// MainWindow.xaml 的交互逻辑
/// </summary>
public partial class MainWindow : Window
{
public static GlobalVariable GlobalVariables { get; set; } = new GlobalVariable();
public MainWindow()
{
InitializeComponent();
// 绑定库
Star.SetBinding(Polygon.FillProperty, new Binding() { Source = GlobalVariables, Path = new PropertyPath("MyStar") });
// 启动线程
new Thread(ThreadLog) { IsBackground = true }.Start();
}
private void ThreadLog()
{
List<string> colors = new List<string>() { "Black", "Yellow", "DarkOrange", "SpringGreen", "Blue" };
while (true)
{
foreach (string color in colors)
{
GlobalVariables.MyStar = color;
Thread.Sleep(1000);
}
}
}
}
public class GlobalVariable : ModelBase
{
private string _MyStar;
/// <summary>
/// MyStar背景色
/// </summary>
public string MyStar
{
set
{
_MyStar = value;
this.OnPropertyChanged("MyStar");
}
get { return _MyStar; }
}
}
[Serializable]
public abstract class ModelBase : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged(string propertyName)
{
if (propertyName != null)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
}
}
MainWindow.xaml.cs 简化代码:
using System;
using System.Collections.Generic;
using System.Threading;
using System.Windows;
using System.Windows.Media;
namespace WpfApp
{
/// <summary>
/// MainWindow.xaml 的交互逻辑
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
// 启动线程
new Thread(ThreadLog) { IsBackground = true }.Start();
}
private void ThreadLog()
{
List<Brush> colors = new List<Brush>() { Brushes.Black, Brushes.Yellow, Brushes.DarkOrange, Brushes.SpringGreen, Brushes.Blue };
while (true)
{
foreach (Brush color in colors)
{
Application.Current.Dispatcher.BeginInvoke((Action)delegate ()
{
Star.Fill = color;
});
Thread.Sleep(1000);
}
}
}
}
}