根据公网IP获取地址信息 - (第三十九讲)
视频讲解如下:
下面给大家讲讲如何通过公网IP获取该IP的地址信息,获取方式有三种,由于代码片段就一个cs文件,我这里就不提供工程包下载地址了,后面直接提供完整的源码。
1、通过百度地图API获取
百度地图的API可以免费申请,但是有次数限制,如果想不受次数限制,就需要购买了。
申请地址:https://lbsyun.baidu.com/apiconsole/key#/home
进入之后界面如下:
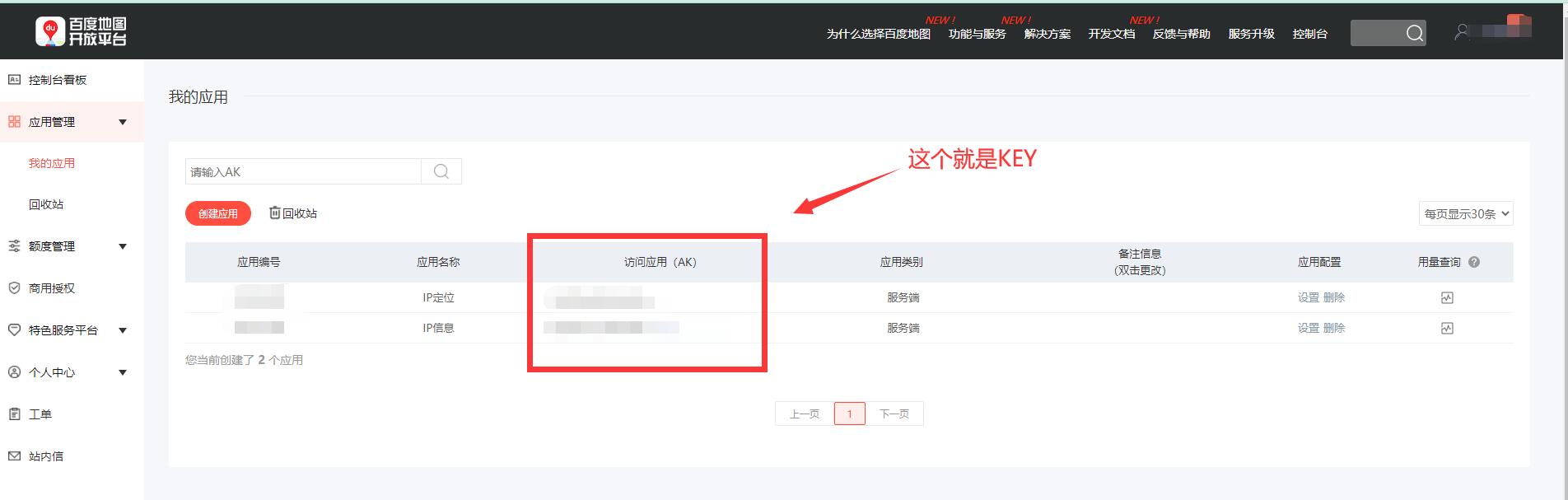
API的调用格式如下:
http://api.map.baidu.com/location/ip?ip=[IP]&ak=[key]
百度地图的API,相对搜狐和IP138,能够提供的信息更全一些,其中包括省份、国籍、经纬度等等,但是只能获取国内的信息,国外的IP好像获取不了。
国内IP测试结果如下:

国外IP测试结果如下:

2、通过搜狐API获取
搜狐API无需提供IP,它是直接获取访问用户当前的地理信息,信息很少,只有公网IP、邮政编码、省份信息,局限性比较大。
http://pv.sohu.com/cityjson?ie=utf-8
测试结果如下:

3、通过ip138 API获取
IP138应该算是比较专业的,国内外的信息都能获取,但是只有一次试用机会,没办法一直试用,而且只能手机号注册,由于我已经试用多了,所以这里没办法演示了,只能告诉大家怎么调用了。
API的调用格式如下:
https://api.ip138.com/ip/?ip=[IP]&datatype=txt&token=[KEY]
API地址申请如下:
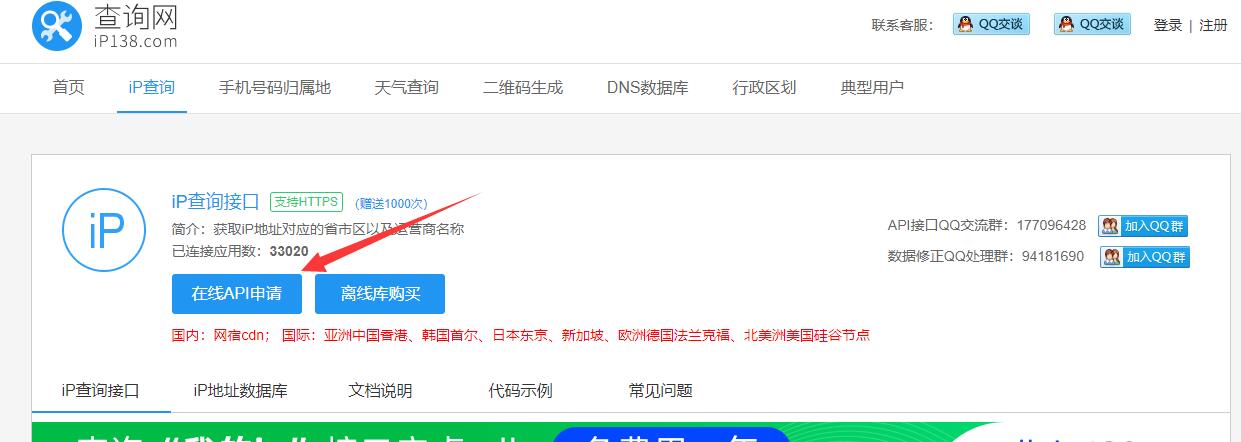
测试代码运行结果如下:
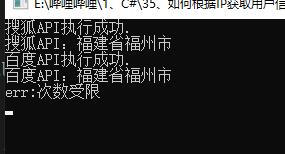
测试代码如下:
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net;
using System.Text;
namespace ConsoleApp
{
class Program
{
public static void Main(string[] args)
{
SoHuIpData a = GetUserAddr1();
if (a != null)
{
Console.WriteLine("搜狐API:" + a.cname);
}
UserIpData b = GetUserAddr2("211.97.116.172");
if (b != null)
{
Console.WriteLine("百度API:" + b.content.address);
}
List<string> c = GetUserAddr3("211.97.116.172");
if (c != null)
{
foreach (string s in c)
Console.WriteLine(s);
}
Console.Read();
}
/// <summary>
/// 搜狐API:http://pv.sohu.com/cityjson?ie=utf-8
/// </summary>
public static SoHuIpData GetUserAddr1()
{
SoHuIpData pack = new SoHuIpData();
try
{
Uri httpURL = new Uri("http://pv.sohu.com/cityjson?ie=utf-8");
HttpWebRequest httpReq = (HttpWebRequest)WebRequest.Create(httpURL);
HttpWebResponse httpResp = (HttpWebResponse)httpReq.GetResponse();
Stream respStream = httpResp.GetResponseStream();
StreamReader respStreamReader = new StreamReader(respStream, Encoding.UTF8);
string strBuff = respStreamReader.ReadToEnd().Split('=')[1].Trim().Split(';')[0];
pack = JsonConvert.DeserializeObject<SoHuIpData>(strBuff);
if (pack != null)
{
Console.WriteLine("搜狐API执行成功.");
}
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
return (pack == null) ? null : pack;
}
/// <summary>
/// 利用百度地图IP地址信息
/// 申请KEY:https://lbsyun.baidu.com/apiconsole/key#/home
/// API格式:http://api.map.baidu.com/location/ip?ip=[IP]&ak=[key]
/// </summary>
public static UserIpData GetUserAddr2(string ip)
{
UserIpData pack = new UserIpData();
try
{
Uri httpURL = new Uri("http://api.map.baidu.com/location/ip?ip=" + ip + "&ak=[KEY]");
HttpWebRequest httpReq = (HttpWebRequest)WebRequest.Create(httpURL);
HttpWebResponse httpResp = (HttpWebResponse)httpReq.GetResponse();
Stream respStream = httpResp.GetResponseStream();
StreamReader respStreamReader = new StreamReader(respStream, Encoding.UTF8);
string strBuff = respStreamReader.ReadToEnd();
pack = JsonConvert.DeserializeObject<UserIpData>(strBuff);
if(pack.address != null)
{
Console.WriteLine("百度API执行成功.");
}
}
catch { }
return (pack.address == null) ? null : pack;
}
/// <summary>
/// ip138 的一个API接口,免费的有次数限制.
/// https://api.ip138.com/ip/?ip=[IP]&datatype=txt&token=[KEY]
/// </summary>
/// <param name="ip"></param>
/// <returns></returns>
public static List<string> GetUserAddr3(string ip = null)
{
try
{
string token = 这是KEY;
string url = string.Format("https://api.ip138.com/ip/?ip={0}&datatype={1}&token={2}", ip, "txt", token);
using (WebClient client = new WebClient())
{
client.Encoding = Encoding.UTF8;
string str = client.DownloadString(url);
if (!str.Contains("err"))
{
List<string> data = str.Split('\t')[1].Split(' ').ToList();
data.Remove(data[data.Count - 1]);
string a = "";
foreach (string s in data) a += (s + " ");
return a.Trim().Split(' ').ToList();
}
else Console.WriteLine(str);
}
}
catch { }
return null;
}
}
#region 搜狐API解析结果
public class SoHuIpData
{
public string cip { get; set; }
public string cid { get; set; }
public string cname { get; set; }
}
#endregion
#region 百度API解析结果
public class UserIpData
{
public string address { get; set; }
public contents content { get; set; } = new contents();
public string state { get; set; }
}
public class contents
{
public address_details address_detail { get; set; }
public points point { get; set; }
public string address { get; set; }
public string status { get; set; }
}
public class address_details
{
public string province { get; set; }
public string city { get; set; }
public string district { get; set; }
public string street_number { get; set; }
public string adcode { get; set; }
public string street { get; set; }
public string city_code { get; set; }
}
public class points
{
public double x { get; set; }
public double y { get; set; }
}
#endregion
}