打开与保存文件 - (第三十六讲)
视频讲解如下:
源码下载,提取码:fyi3
https://pan.baidu.com/s/1OvRbkERvHJCjX0Mpla8zEw
下面演示在WinForm环境下如何实现文件的打开、保存、另存,三种操作的。
首先呢,需要在工具栏里面添加openFileDialog控件。
演示效果如下
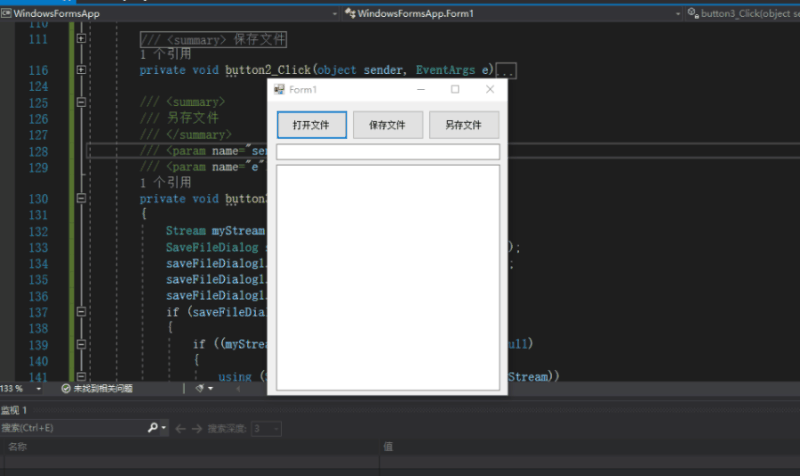
演示代码如下
using System;
using System.IO;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApp
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
/// <summary>
/// 判断文件格式
/// </summary>
/// <param name="filePath"></param>
/// <returns></returns>
private bool IsAllowedExtension(string filePath)
{
FileStream stream = new FileStream(filePath, FileMode.Open, FileAccess.Read);
BinaryReader reader = new BinaryReader(stream);
string fileclass = "";
try
{
for (int i = 0; i < 2; i++)
{
fileclass += reader.ReadByte().ToString();
}
}
catch
{
stream.Close();
throw;
}
if (fileclass == "230191" || fileclass == "239187")
{
stream.Close();
return true;
}
else
{
stream.Close();
return false;
}
}
/// <summary>
/// 读取文件
/// </summary>
/// <param name="path"></param>
/// <returns></returns>
public byte[] ReadFile(string path)
{
try
{
if (path == "") return null;
if (File.Exists(path))
{
if (true == IsAllowedExtension(path))
{
try
{
using (FileStream fs = new FileStream(path, FileMode.Open))
{
byte[] arrFile = new byte[30000];
int num = 29999;
int length = fs.Read(arrFile, 0, arrFile.Length);
if (length != 0)
{
while ((arrFile[num] == 0x00) && (num > 0)) num--;
byte[] oData = new byte[num + 1];
Array.Copy(arrFile, oData, num + 1);
if (oData.Length == 0) return null;
return oData;
}
}
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
}
}
}
catch { }
return null;
}
/// <summary>
/// 打开文件
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void button1_Click(object sender, EventArgs e)
{
this.openFileDialog1.FileName = "";
this.openFileDialog1.Filter = "*.txt|*.txt|所有文件(*.*)|*.*";
if (this.openFileDialog1.ShowDialog() == DialogResult.OK)
{
textBox2.Text = this.openFileDialog1.FileName;
// 读取文件
byte[] arrFile = ReadFile(this.openFileDialog1.FileName);
// Byte[] 转 string
textBox1.Text = System.Text.Encoding.UTF8.GetString(arrFile);
}
}
/// <summary>
/// 保存文件
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void button2_Click(object sender, EventArgs e)
{
// 第二个参数:false是覆盖保存,true是追加保存
StreamWriter sw = new StreamWriter(textBox2.Text, false, Encoding.UTF8);
sw.Write(textBox1.Text);
sw.Close();
MessageBox.Show("保存成功!", "提示");
}
/// <summary>
/// 另存文件
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void button3_Click(object sender, EventArgs e)
{
Stream myStream;
SaveFileDialog saveFileDialog1 = new SaveFileDialog();
saveFileDialog1.Filter = "txt files (*.txt)|*.txt";
saveFileDialog1.FilterIndex = 2;
saveFileDialog1.RestoreDirectory = true;
if (saveFileDialog1.ShowDialog() == DialogResult.OK)
{
if ((myStream = saveFileDialog1.OpenFile()) != null)
{
using (StreamWriter sw = new StreamWriter(myStream))
{
sw.Write(textBox1.Text);
}
myStream.Close();
MessageBox.Show("保存成功!", "提示");
}
}
}
}
}
另一种保存文件的方式
/// <summary>
/// 另一种保存文件的方式(追加写)
/// </summary>
/// <param name="data"></param>
public void WriteFile(string data)
{
try
{
if (!Directory.Exists(Environment.CurrentDirectory))
{
Directory.CreateDirectory(Environment.CurrentDirectory);
}
string FileName = Environment.CurrentDirectory + DateTime.Now.ToString("yyyyMMddhh") + "_log.txt";
using (StreamWriter file = new StreamWriter(FileName, true))
{
file.Write(data);
file.Close();
}
}
catch { }
}