重写TabControl控件实现拖拽 - (第十四讲)
视频讲解如下:
网盘下载,提取码:pt2b
https://pan.baidu.com/s/1C2ri-X89szdMtxfgc-feGg
这里给大家讲解一下如何重写TabControl控件实现拖拽
拖拽效果演示如下:
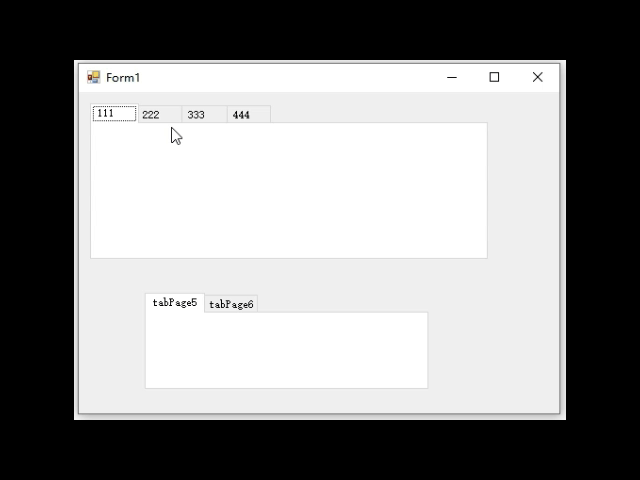
using System;
using System.Collections.Generic;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp
{
/// <summary>
/// 重写控件,实现拖拽功能
/// </summary>
public class MyTabControl : System.Windows.Forms.TabControl
{
public MyTabControl()
{
}
protected override void OnMouseDown(MouseEventArgs e)
{
base.OnMouseDown(e);
TabPage tabPage = GetTabPageByTab(new Point(e.X, e.Y));
if (tabPage != null)
{
this.DoDragDrop(tabPage, DragDropEffects.All);
}
}
private TabPage GetTabPageByTab(Point point)
{
for (int i = 0; i < this.TabPages.Count; i++)
{
if (GetTabRect(i).Contains(point))
{
return this.TabPages[i];
}
}
return null;
}
protected override void OnDragOver(DragEventArgs e)
{
base.OnDragOver(e);
TabPage source = (TabPage)e.Data.GetData(typeof(TabPage));
if (source != null)
{
TabPage target = GetTabPageByTab(PointToClient(new Point(e.X, e.Y)));
if (target != null)
{
e.Effect = DragDropEffects.Move;
MoveTabPage(source, target);
}
else
{
e.Effect = DragDropEffects.None;
}
}
else
{
e.Effect = DragDropEffects.None;
}
}
private void MoveTabPage(TabPage source, TabPage target)
{
if (source == target)
return;
int targetIndex = -1;
List<TabPage> lstPages = new List<TabPage>();
for (int i = 0; i < this.TabPages.Count; i++)
{
if (this.TabPages[i] == target)
{
targetIndex = i;
}
if (this.TabPages[i] != source)
{
lstPages.Add(this.TabPages[i]);
}
}
this.TabPages.Clear();
this.TabPages.AddRange(lstPages.ToArray());
this.TabPages.Insert(targetIndex, source);
this.SelectedTab = source;
}
}
}