DES数据加密与解密 - (第三十四讲)
视频讲解如下:
源码下载,提取码:52oi
https://pan.baidu.com/s/1TTLZAQ7sfFIInIsEjv2yGQ
下面提供了一种DES的数据加密与解密的算法。
演示效果如下
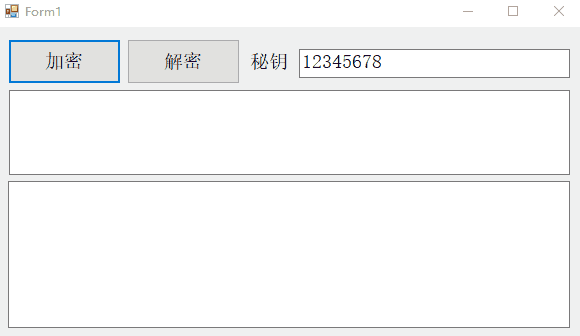
using System;
using System.IO;
using System.Security.Cryptography;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApp
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
/// <summary>
/// DES数据加密
/// </summary>
/// <param name="targetValue">目标值</param>
/// <param name="key">密钥</param>
/// <returns>加密值</returns>
public string Encrypt(string targetValue, string key)
{
//实例化加密类对象
DESCryptoServiceProvider des = new DESCryptoServiceProvider();
//定义字节数组用来存放密钥 GetBytes方法用于将字符串中所有字节编码为一个字节序列
byte[] arr_key = Encoding.UTF8.GetBytes(key.Substring(0, 8));
//!!!DES加密key位数只能是64位,即8字节
//注意这里用的编码用当前默认编码方式,不确定就用Default
byte[] arr_str = Encoding.UTF8.GetBytes(targetValue);//定义字节数组存放要加密的字符串
MemoryStream ms = new MemoryStream();//实例化内存流对象
//创建加密流对象,参数 内存流/初始化向量IV/加密流模式
CryptoStream cs = new CryptoStream(ms, des.CreateEncryptor(arr_key, arr_key), CryptoStreamMode.Write);
//需加密字节数组/offset/length,此方法将length个字节从 arr_str 复制到当前流。0是偏移量offset,即从指定index开始复制。
cs.Write(arr_str, 0, arr_str.Length);
cs.Close();
string str_des = Convert.ToBase64String(ms.ToArray());
return str_des;
}
/// <summary>
/// DES数据解密
/// </summary>
/// <param name="targetValue"></param>
/// <param name="key"></param>
/// <returns></returns>
public string Decrypt(string targetValue, string key)
{
//定义字节数组用来存放密钥 GetBytes方法用于将字符串中所有字节编码为一个字节序列
byte[] arr_key = Encoding.UTF8.GetBytes(key.Substring(0, 8));
MemoryStream ms = new MemoryStream();
//注意这里仍要将密文作为base64字符串处理获得数组,否则报错
byte[] arr_des = Convert.FromBase64String(targetValue);
//解密方法定义加密对象
DESCryptoServiceProvider des = new DESCryptoServiceProvider();
CryptoStream cs = new CryptoStream(ms, des.CreateDecryptor(arr_key, arr_key), CryptoStreamMode.Write);
cs.Write(arr_des, 0, arr_des.Length);
cs.FlushFinalBlock();
cs.Close();
return Encoding.UTF8.GetString(ms.ToArray());
}
private void button1_Click(object sender, EventArgs e)
{
textBox2.Text = Encrypt(textBox1.Text, textBox3.Text);
}
private void button2_Click(object sender, EventArgs e)
{
textBox1.Text = Decrypt(textBox2.Text, textBox3.Text);
}
}
}